Your cart is currently empty!
Bulk variable subscription pricing
Changes subscription variation prices by a multiplier in batches of products. Located in WooCommerce > Settings > Products > Bulk variable subscription pricing.
// Add Settings Tab
add_filter( 'woocommerce_get_sections_products', function( $sections ) {
$sections['ccom_bulkpricing'] = 'Bulk variable subscription pricing';
return $sections;
} );
// Add Settings Fields
add_filter( 'woocommerce_get_settings_products',
function( $settings, $current_section ) {
// Only This Section
if ( $current_section != 'ccom_bulkpricing' ) {
return $settings;
}
// Return Settings Array
return [
// Add Section Title
[ 'id' => 'ccom_bvsp_title', 'name' => 'Bulk Variable Subscription Pricing', 'type' => 'title' ],
// Settings
[
'id' => 'ccom_bvsp_percentage', 'name' => 'Multiplier', 'type' => 'text',
'desc' => 'Enter a positive or negative multiplier.',
'desc_tip' => 'Example: To increase prices by 10% enter 1.1 in this field and empty the below field Basing.',
],
[
'id' => 'ccom_bvsp_field', 'name' => 'Basing', 'type' => 'text',
'desc' => 'Which custom product field to base price on? Leave blank to use the current variation price.',
'desc_tip' => ' Example: _retail_price field then set the above Multiplier field to 0.10 for 10% of retail price.',
],
[
'id' => 'ccom_bvsp_signup', 'name' => 'Signup fee', 'type' => 'checkbox',
'desc' => 'Check to set the signup fee to the same amount, otherwise will set it to zero.',
],
[
'id' => 'ccom_bvsp_attr_name', 'name' => 'Attribute name', 'type' => 'text',
'desc' => 'Which subscription variation attribute shall we affect?',
'desc_tip' => 'Example: attribute_pa_rental-period',
],
[
'id' => 'ccom_bvsp_attr_value', 'name' => 'Attribute value', 'type' => 'text',
'desc' => 'Which value from the above selected attribute shall we affect?',
'desc_tip' => 'Example: rent-monthly or rent-1-7-days',
],
// Add Run Now Field
[
'id' => 'ccom_bvsp_run',
'name' => 'Run Now',
'type' => 'textarea',
'placeholder' =>
'Updates 25 products at a time.'
. ' Results appear inside this box.'
. ' When done box it will read [EOF].',
'desc' => '
<p>
<a href="#" class="button" id="ccom_bulkpricing_button">
Run variable subscription price updater
</a>
</p>
<p>
If you have changed any values on this page,
please click Save changes button before running.
</p>
',
],
// End Settings Section
[ 'id' => 'ccom_bvsp_section_end', 'type' => 'sectionend' ],
];
}, 10, 2 );
// AJAX Handler
add_action( 'wp_ajax_ccom_bulkpricing', function() {
// Get Set Of Parent / Variable Products
$offset = empty( $_POST['offset'] ) ? 0 : intval( $_POST['offset'] );
$args = [
'limit' => 25,
'offset' => $offset,
'orderby' => 'ID',
'order' => 'ASC',
'status' => 'publish',
];
$products = wc_get_products( $args );
if( ! $products ) {
wp_die();
}
// Loop Products
foreach( $products as $product ) {
// Print Product ID
printf( '[%d] ', $product->get_id() );
// Variations Only
if( ! $product->is_type( 'variable' ) ) {
continue;
}
// Get Variations
$variations = $product->get_available_variations();
foreach( $variations as $variation ) {
// Update Signup Fee For Monthly Variation
foreach( $variation['attributes'] as $attribute_name => $attribute_value ) {
if(
$attribute_name == get_option( 'ccom_bvsp_attr_name' )
&& $attribute_value == get_option( 'ccom_bvsp_attr_value' )
) {
$variation_id = $variation['variation_id'];
$product_variation = wc_get_product( $variation_id );
printf( '(%d) ', $variation_id );
// Get Price
$price = ( float ) $product_variation->get_price();
$ccom_bvsp_field = get_option( 'ccom_bvsp_field' )
? $product->get_meta(
get_option( 'ccom_bvsp_field' ), true
) : $price;
if( ! $ccom_bvsp_field ) { continue; }
$new_price = number_format(
$ccom_bvsp_field * get_option( 'ccom_bvsp_percentage' ), 2
);
// Set Price
$product_variation->update_meta_data( '_regular_price', $new_price );
$product_variation->update_meta_data( '_price', $new_price );
$product_variation->update_meta_data( '_subscription_price', $new_price );
$product_variation->update_meta_data(
'_subscription_sign_up_fee',
get_option( 'ccom_bvsp_signup' ) == 'yes' ? $new_price : 0
);
$product_variation->save();
wc_delete_product_transients( $variation_id );
}
}
}
// Sync Parent Price Ranges
wc_delete_product_transients( $product->get_id() );
$product->variable_product_sync();
}
// Exit
wp_die();
} );
// jQuery
add_action( 'admin_enqueue_scripts', function( $page ) {
// Only Load On Our Settings Page
if( $page != 'woocommerce_page_wc-settings' ) { return; }
if( empty( $_REQUEST['section'] ) || $_REQUEST['section'] != 'ccom_bulkpricing' ) {
return;
}
ob_start();
?>
<script type="text/javascript">
var ccom_bulkpricing_offset = 0;
jQuery( document ).ready( function( $ ) {
$( "#ccom_bulkpricing_button" ).click( function() {
ccom_bulkpricing( $ );
return false;
} );
} );
function ccom_bulkpricing( $ ) {
var data = {
"action": "ccom_bulkpricing",
"offset": ccom_bulkpricing_offset
};
$.post( ajaxurl, data, function( response ) {
// Handle EOF Or Error
if( response == "" ) {
$( "textarea#ccom_bvsp_run" ).append( " [EOF] " );
return;
}
// Display Page Processed
$( "textarea#ccom_bvsp_run" ).append( " [" + ccom_bulkpricing_offset + "] " );
// Advance
ccom_bulkpricing_offset += 25;
ccom_bulkpricing( $ );
} );
}
</script>
<?php
$js = ob_get_clean();
wp_add_inline_script( 'jquery', $js );
} );
Instructions for Bulk variable subscription pricing
- Log into a staging or locally hosted clone of your site.
- Install and activate Code Snippets plugin.
- WP Admin > Snippets > Add New
- Copy and paste the code from the section above.
- Check to ensure formatting came over properly.
- Customize the code as desired.
- Add a meaningful title.
- Select whether to run on front-end or back-end (or both).
- Click “Save and Activate”.
- Test your site to ensure it works.
- Disable if any problems, or recover.
- Repeat for live environment.
Need help modifying Bulk variable subscription pricing?
Contact me. I can help with fitting projects or refer to my partner.
License
All code snippets are licensed GPLv2 (or later) matching WordPress licensing.
Except when otherwise stated in writing the copyright holders and/or other parties provide the program as-is without warranty of any kind, either expressed or implied, including, but not limited to, the implied warranties of merchantability and fitness for a particular purpose.
Disclaimer of warranty
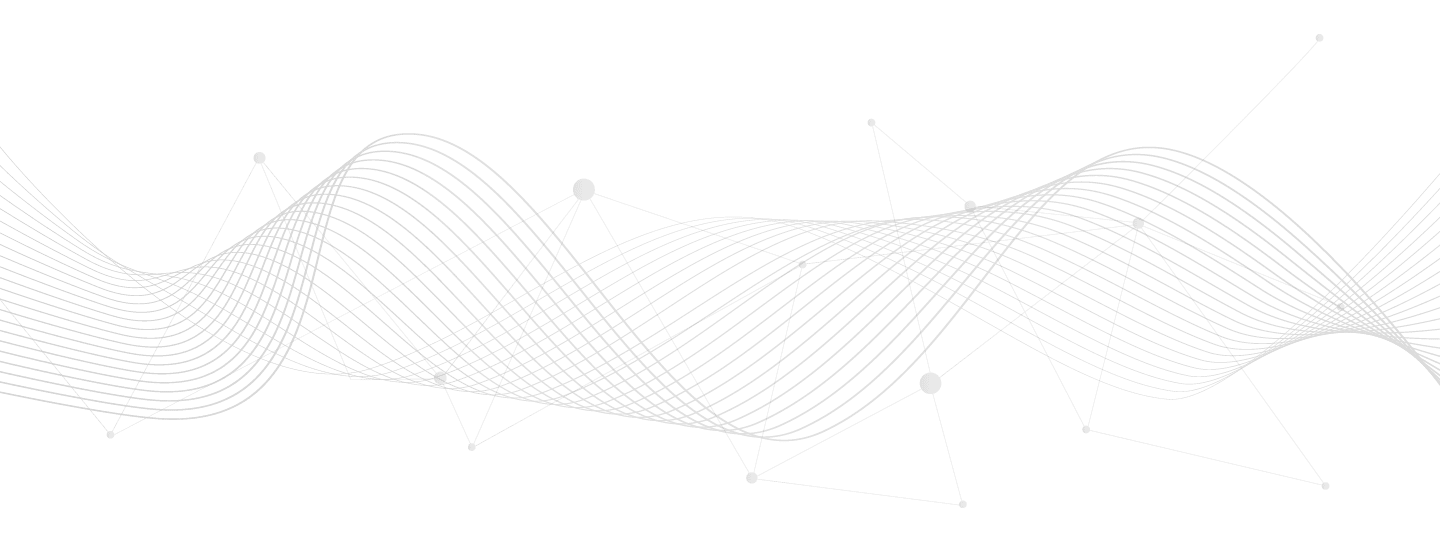